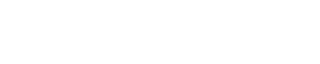
-
Python Caveats #1: How to put multiline comments in Python dict?
How to put multiline comments in python dict? Should we use triple quotes style or multiline hashes?
-
Collection of Python posts for beginners
I took MITx 6.00x on edX in 2012 and we were taught basics of Python along-with programming in general. While revisiting the videos in the end, I thought of creating a quick guide for myself and everyone else.
-
Python: Dictionary and its properties
Dictionary is a generalization of a List. Dictionary stores (key, value) pair for easier value access in future using the key. We see how it is different from a list and its properties.
-
Python: Tuples and Basic Operations on Tuples
What is a tuple? How is it different from a list? And some basic methods on Tuples
-
Python: Lists and List methods
Declaring, Accessing, Slicing, Deleting and Updating Lists and its elements in Python
-
Python: Linear Search v/s Bisection (Binary) Search
When it comes to searching an element within a list of elements, our first approach is searching sequentially through the list. Let's take a look at a better method, Binary Search
-
Basics of Recursion with an example in Python
Recursion is a technique of finding solutions to larger problems using known solution of smaller problems. For me atleast, it was hard to get the understanding of recursion. In this post we see an iterative and recursive version of one problem in programming.
-
Python: Finding Square Root using Guess & Check Algorithm
Guess and Check is one of the most common methods of finding solution to any problem. We will see how it can be used to find a close approximation of square root of any number
-
Python: Perform repetitive tasks using Iteration or Loop
For and While loop constructs in Python enable us to perform repetitive tasks or help us iterate over a string or a list or any iterable object
-
Python: Branching code flow using if - else - elif
If this Then that! Python provides us with if, else and elif to add conditions in code and branch it like a tree
-
Python: Strings and it's frequently used methods
Accessing string characters via index, string slicing and frequently used str methods such as find, replace. How to read input from Standard Input STDIN
-
Python: Arithmetic and Logical Operators
How to perform arithmetic and logical operations on Python variables of different types
-
Python: Objects and Types
Everything in Python is an object! Every object in Python has a type. This post discusses some of them with examples
-
Python: Syntax, Static Semantics, Semantics of a Language
Before we begin with any language, it's important to know the aspects of the language. What is Syntax? Semantics? Static Semantics? This post explain these terms with examples.