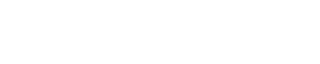
-
Extended Euclid Algorithm to find GCD and Bézout's coefficients
We will see how to use Extended Euclid's Algorithm to find GCD of two numbers. It also gives us Bézout's coefficients (x, y) such that ax + by = gcd(a, b). We will discuss and implement all of the above problems in Python and C++
-
How to find Multiplicative Inverse of a number modulo M?
What is Multiplicative Inverse? What is Modular Multiplicative Inverse? How to find Modular Multiplicative Inverse? How to find Multiplicative Inverse of a number modulo M i.e. under M? How to find Modular Multiplicative Inverse in an efficient way? We will discuss and implement all of the above problems in Python and C++
-
Fastest way to check if a number is prime or not - Python and C++ Code
How to check if a given number is a prime number? What is the most efficient way to check if a number is prime or not? How to find prime numbers in a given list of numbers? We will discuss and implement all of the above problems in Python and C++
-
Most efficient way to find factors of a number - C++ and Python Code
First, we will see how to find all factors of a number using brute force. Then we will improve upon that to find all factors of a number using the most efficient method and code it in C++ as well as Python
-
Hobbyist Competitive Programmer to Software Developer at HackerEarth
During four years of Computer Engineering, I used to think about what I should pursue as professional career. Loved designing graphic posters, thoroughly enjoyed Competitive Programmer and it was immensely satisfying to build software products to solve real world problems. So much dilemma and I ended up being a Software Engineer at HackerEarth
-
What is Memoization? What is Dynamic Programming? Let's take an example
Memoization or Dynamic Programming is a technique of remembering solutions to sub-problems which will help us solve a larger problem.
-
Greatest Common Divisor of a list of numbers - C++ and Python Implementation
First, we will see how to find GCD of two numbers. It is also known as Highest Common Factor - HCF. Then we will move on to find the GCD of a list of numbers and code it in both C++ as well as Python
-
Fast Power Algorithm - Exponentiation by Squaring - C++ and Python Implementation
We know how to find 2 raised to the power 10. But what if we have to find 2 raised to the power very large number such as 1000000000? We discuss how to find solution to such a problem using an fast, efficient algorithm
-
How to compute Time Complexity or Order of Growth of any program
Time Complexity gives us an idea of running time of any program w.r.t. the size of input fed to the program. Order of Growth is just another word for Time Complexity.
-
Python: Linear Search v/s Bisection (Binary) Search
When it comes to searching an element within a list of elements, our first approach is searching sequentially through the list. Let's take a look at a better method, Binary Search
-
Basics of Recursion with an example in Python
Recursion is a technique of finding solutions to larger problems using known solution of smaller problems. For me atleast, it was hard to get the understanding of recursion. In this post we see an iterative and recursive version of one problem in programming.